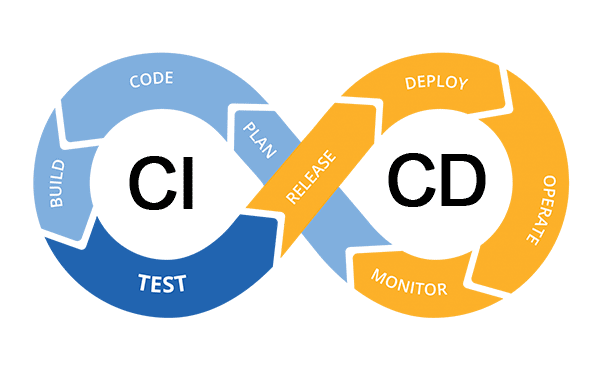
CI/CD Pipeline For a Lambda Function
This project allowed me to discover GitHub Actions and AWS Together. It was very interesting and surprisingly very simple to setup.
1 — Overview
2 — Steps
3 — Verification
1 - Overview
The goal of this project is to update an existing lambda Function when code is push in a repository. Secrets will be used to authenticate github actions to AWS to deploy our lambda function.
Runner :
- Checks out the code from the repository
- Setup python envrionement and install dependencies
- Configure aws credentials
- Package up our lambda
- Deploy the lambda to aws
We will create the lambda from aws console to speed up things.
2 - Steps
1 - Create a new GitHub repo
Using GitHub website.
2 - Create a new actions secret
We need to securely store our aws credentials to authenticate our pipeline with aws.
3 - Create the lambda function
4 - Add code to our repo for the lambda
Initially, the code of the lambda simply print “Hello from Lambda” in response of HTTP Request. We want to change this behavior using our pipeline to update the code.
import json
def lambda_handler(event, context):
# TODO implement
return {
'statusCode': 200,
'body': json.dumps('Hello from Lambda!')
}
Best practice is to always include a requirements.txt file for python dependencies.
We add the code for our pipeline in .github/workflows/lambda.yaml
name: Deploy AWS Lambda
on:
push:
branches:
- main
paths:
- "lambda/*"
jobs:
deploy-lambda: # Job name
runs-on: ubuntu-latest # Specify the runner
steps:
# 1 - Checkout and get the code in the runner environment
- uses: actions/checkout@v2
# 2 - Set up Python environment
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: "3.12"
# 3 - Install dependencies
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r lambda/requirements.txt
# 4 - Setup AWS credentials
- name: Configure AWS Credentials
uses: aws-actions/configure-aws-credentials@v2
with:
aws-access-key-id: ${{ secrets.AWS_ACCESS_KEY_ID }}
aws-secret-access-key: ${{ secrets.AWS_SECRET_ACCESS_KEY }}
aws-region: eu-west-3
# 5 - Zips the lambda code and updates the lambda function
- name: Deploy Lambda
run: |
cd lambda
zip -r lambda.zip .
aws lambda update-function-code --function-name my-test-cicd-lambda --zip-file fileb://lambda.zip
We can update our code to :
import json
def lambda_handler(event, context):
# TODO implement
return {
'statusCode': 200,
'body': json.dumps('Hello from Lambda changes made from vscode!')
}
5 - Commit & Push
We commit and pushes the changes which will trigger the pipeline :
3 - Verification
Then if we go to the aws console we can seen that the code has been updated :
It’s a success, we updated our function using GitHub Actions Pipeline. We can now delete our function.